The popular web application framework, referred to as Rails or Ruby on Rails, is lauded for its efficient and elegant approach to web application building.
An integral component of this framework is routing. Routing defines how incoming HTTP requests are managed by the application.
Mastering the configuration of routes is necessary for creating a sophisticated and useful Rails application. In this piece, we’ll take a deep dive into the significant role of defining and configuring routes for a Ruby on Rails application, complete with examples and a step-by-step guide.
Creating a Rails Application
You need to create a Ruby on Rails application before you can start routing. In case you haven’t already, here’s a command to start a new Rails application.
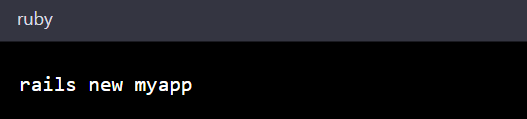
This command will generate the necessary files and directory structure to start building your application.
Routes in a Rails Application
The crucial config/routes.rb file defines the routes within a Rails application. It plays a pivotal role in determining which actions and controllers incoming requests get directed to.
1. Basic Routing
Based on the RESTful design, routing in Rails is fundamentally anchored on the standard CRUD operations. Therefore, routes are configured to operate accordingly. Here is a simple illustration of setting up routes for a given resource.

In this example, we define routes for the Article resource, which includes routes for listing, creating, reading, updating, and deleting articles.
2. Custom Routes
While Rails provides conventional RESTful routes, you can also define custom routes to handle specific actions or non-standard paths. For instance:
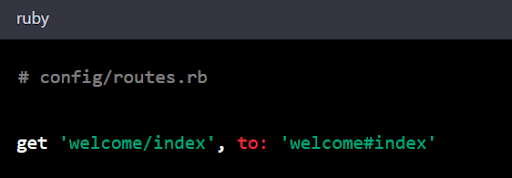
This route maps the URL path /welcome/index to the WelcomeController and its index action.
3. Route Parameters
You can capture dynamic segments in the URL and pass them as parameters to your controller actions. For example:

In this case, the :id segment in the URL will be passed to the show action of the ArticlesController.
Route Constraints
Rails allows you to apply constraints to routes based on certain conditions. This is particularly useful for routing to different controllers or actions depending on factors like subdomains or request formats.

In this example, requests with the subdomain ‘api’ will be routed to the Api::ArticlesController.
Namespace vs Scope Routing
When it comes to customising the rails routes, some options like namespace and scope pops up which also has a lot of use case and importance in Rails application
Namespace
The simplest way we can think of “namespace” is url_prefix, it affects the url_helper and invokes actions of controllers which are under the same namespace folder.
namespace :admin do
resources :users
end
This one change results in a lot of advantages from maintaining/organising Api’s to versioning the api’s.
We’ll will end up with the following routes:
Prefix Verb URI Pattern Controller#Action
admin_users GET /admin/users(.:format) admin/users#index
POST /admin/users(.:format) admin/users#create
admin_user GET /admin/users/:id(.:format) admin/users#show
PATCH /admin/users/:id(.:format) admin/users#update
PUT /admin/users/:id(.:format) admin/users#update
DELETE /admin/users/:id(.:format) admin/users#destroy
Scope
It is a bit more complex – but the advantage is that it gives you more options to fine-tune exactly what we want to do.
Three options that scope supports are module, path and as.
module lets us define in which module the controller for the embedded resources will live without affecting the URI Pattern.
scope module: 'admin' do
resources :users
end
Prefix Verb URI Pattern Controller#Action
users GET /users(.:format) admin/users#index
POST /users(.:format) admin/users#create
user GET /users/:id(.:format) admin/users#show
path allows us to set the prefix that will appear in the URL.
scope module: 'admin', path: 'fu' do
resources :users
end
Prefix Verb URI Pattern Controller#Action
users GET /fu/users(.:format) admin/users#index
POST /fu/users(.:format) admin/users#create
Finally, as can be used to change the name of the path method used to identify the resources.
Rails Application Configuration
Apart from defining routes, Rails applications come with various configuration options to customise your application’s behaviour. Here are some key elements:
1. Rails Application Credentials
Rails provides a secure way to manage sensitive information such as API keys and database passwords. You can store and access these credentials using the config/credentials.yml.enc file and the Rails.application.credentials object.
2. Rails Application Controller
Controllers in Rails are responsible for handling incoming requests, interacting with models, and rendering views. Proper controller organisation is essential for a maintainable application structure.
3. Rails Application Record
A Rails model, also known as a record, represents data in your application and is closely tied to the database. ActiveRecord, the default ORM (Object-Relational Mapping) in Rails, simplifies database interactions.
4. Rails Application Config
The config/application.rb file is where you configure various aspects of your Rails application, such as specifying middleware, setting time zone, and defining the default generator behaviour.
Ruby on Rails Application Example
In order to demonstrate these ideas, let’s make a basic example. We’ll lay out the pathways for a blog application that lets users see, add, edit, and remove content.
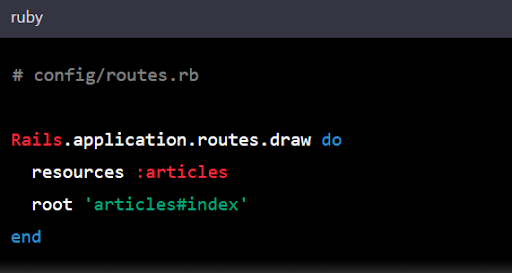
This code sets up routes for the Article resource and makes the index action the root of the application.
Conclusion
In the world of Ruby on Rails application development, defining and configuring routes is a fundamental step that shapes how your application responds to incoming requests.
Understanding RESTful routing, custom routes, and route constraints empowers you to design elegant and efficient web applications.
Alongside route configuration, managing credentials, controllers, records, and other application settings plays a crucial role in building a robust and secure Rails application.
Gaining proficiency in these areas will enable you to create complex web applications and use Ruby on Rails application development services to advance your projects.
You’ll be well on your way to developing robust and intuitive web applications if you always keep in mind to give security and scalability top priority when configuring your Rails application. For more infomation, contact our experts and professionals at Agiledock today.